Hi,
I am a simple JOGL program that displays a red background as seen below.
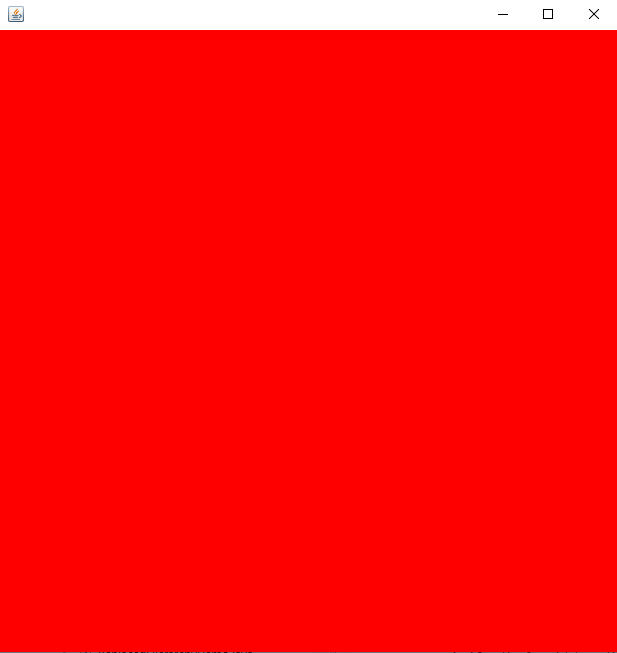
However, if I run the same code on a different machine with Intel UHD Graphics (with NVIDIA Quadro P620), the image is distorted.
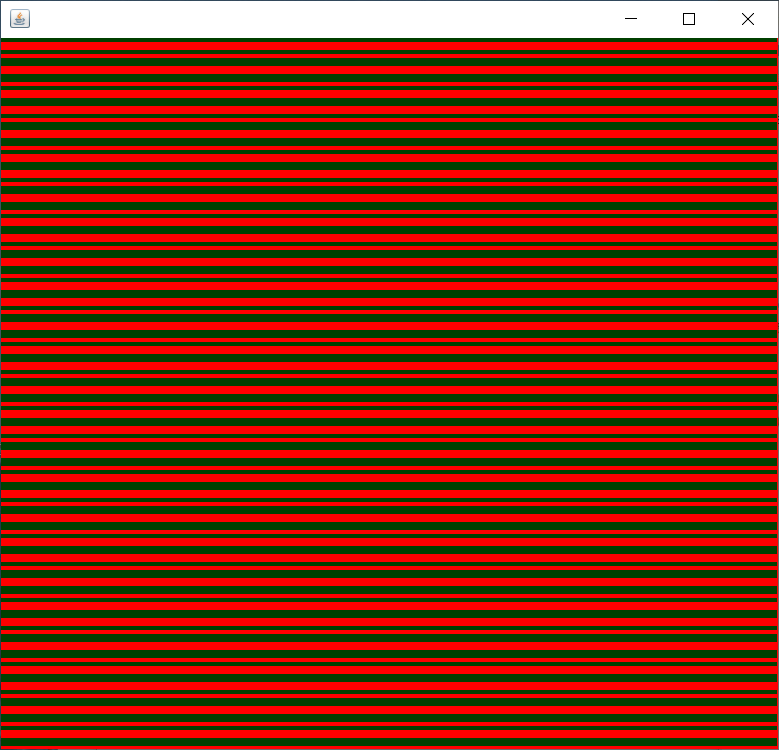
I posted the code below. Please help
public class OpenGLTest implements GLEventListener
{
public static void main(String[] args)
{
GLProfile glp = GLProfile.getDefault();
GLCapabilities caps = new GLCapabilities(glp);
GLCanvas canvas = new GLCanvas(caps);
canvas.setSize(600,600);
canvas.addGLEventListener(new OpenGLTest());
JFrame frame = new JFrame();
frame.setSize(new Dimension(3000, 3000));
frame.add(canvas);
frame.pack();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
@Override
public void display(GLAutoDrawable arg0)
{
// TODO Auto-generated method stub
float[] component = new float[4];
Color.red.getComponents(component);
arg0.getGL().getGL2().glClearColor(component[0], component[1], component[2], 0.0f);
}
@Override
public void dispose(GLAutoDrawable arg0)
{
}
@Override
public void init(GLAutoDrawable arg0)
{
}
@Override
public void reshape(GLAutoDrawable arg0,
int arg1,
int arg2,
int arg3,
int arg4)
{
}
}