Posted by
xghost on
Jul 20, 2014; 11:41am
URL: https://forum.jogamp.org/Need-Help-Solving-OpenGL-GLSL-4-4-Issues-tp4032557p4032596.html
Hey all,
It's been a few days. I've tried a few other things, but have not been successful yet.
I'm posting the code of a simple demo below. It displays a large pixel on the center using a vertex and fragment shader. The background's color alternates, but the large pixel's position and color are hardcoded in the fragment shader.
Here's the thing: No matter what values I enter into the shaders, I cannot alter the pixel's position on screen and I cannot alter the pixel's color. Note that the pixel's black color does NOT match the fragment's RGB component values. (See picture below.)
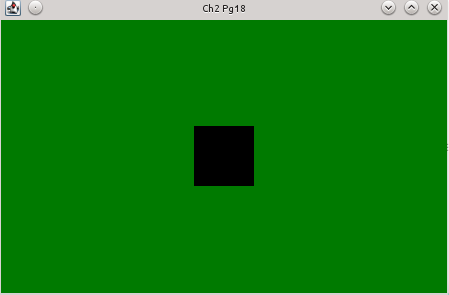
If you think I'm missing something on the OpenGL side of things (i.e. not in the shader code), I'm open to suggestions.
I'd appreciate if someone else could take a few minutes to try this code and report results. Of particular interest is tweaking the hard-coded shader values to see if you can actually manipulate the pixel's color by editing fragment shader values and also its position on the vertex shader.
Note that the shaders seem to be working to some extent because removing the shader program causes the pixel to go away.
Thanks in advance.
PS: Note that there're 2 classes and that you'll also need to update package names, etc.
--------------
/**
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
package ray.cg.demos.demo3;
import java.nio.FloatBuffer;
import javax.media.opengl.GL4;
import javax.media.opengl.GLAutoDrawable;
import ray.cg.lib.DemoFrame;
import com.jogamp.opengl.util.Animator;
public class Demo3 extends DemoFrame {
private static final long serialVersionUID = 1L;
private int renderingProgram;
private final int[] vertexArray;
public Demo3() {
super("Ch2 Pg18");
super.initOpenGL();
setVisible(true);
vertexArray = new int[1];
Animator animator = new Animator(canvas);
animator.start();
}
@Override
public void init(GLAutoDrawable drawable) {
GL4 gl = (GL4) drawable.getGL();
renderingProgram = createRenderingProgram(gl);
gl.glGenVertexArrays(vertexArray.length, vertexArray, 0);
gl.glBindVertexArray(vertexArray[0]);
}
@Override
public void display(GLAutoDrawable drawable) {
GL4 gl = (GL4) drawable.getGL();
FloatBuffer color = FloatBuffer.allocate(4);
color.put(0, (float) (Math.sin(System.currentTimeMillis()/100.0)*0.5 + 0.5));
color.put(1, (float) (Math.cos(System.currentTimeMillis()/100.0)*0.5 + 0.5));
color.put(2, 0.0f);
color.put(3, 1.0f);
gl.glClearBufferfv(GL4.GL_COLOR, 0, color);
gl.glUseProgram(renderingProgram);
gl.glPointSize(60.0f);
gl.glDrawArrays(GL4.GL_POINTS, 0, 1);
}
private int createRenderingProgram(GL4 gl) {
String[] vertexShaderSource = new String[] {
"#version 440 core \n",
" \n",
"void main(void) \n",
"{ \n",
" gl_Position = vec4(0.0, 0.0, 0.5, 1.0); \n",
"} \n"
};
String[] fragmentShaderSource = new String[] {
"#version 440 core \n",
" \n",
"out vec4 color; \n",
" \n",
"void main(void) \n",
"{ \n",
" color = vec4(0.0, 0.8, 1.0, 1.0); \n",
"} \n"
};
int vertexShader = gl.glCreateShader(GL4.GL_VERTEX_SHADER);
gl.glShaderSource(vertexShader, 1, vertexShaderSource, null);
gl.glCompileShader(vertexShader);
int fragmentShader = gl.glCreateShader(GL4.GL_FRAGMENT_SHADER);
gl.glShaderSource(fragmentShader, 1, fragmentShaderSource, null);
gl.glCompileShader(fragmentShader);
int program = gl.glCreateProgram();
gl.glAttachShader(program, vertexShader);
gl.glAttachShader(program, fragmentShader);
gl.glLinkProgram(program);
gl.glDeleteShader(vertexShader);
gl.glDeleteShader(fragmentShader);
return program;
}
public static void main(String[] args) {
new Demo3();
}
@Override
public void dispose(GLAutoDrawable drawable) {}
@Override
public void reshape(GLAutoDrawable drawable, int x, int y, int width,
int height) {}
}
---------------
/**
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
package ray.cg.lib;
import javax.media.opengl.GLEventListener;
import javax.media.opengl.awt.GLCanvas;
import javax.swing.JFrame;
import javax.swing.WindowConstants;
public abstract class DemoFrame extends JFrame implements GLEventListener {
private static final long serialVersionUID = 1L;
protected GLCanvas canvas;
public DemoFrame(String title) {
// XXX: Do NOT setup the GLCanvas in the ctor. See below.
setTitle(title);
setSize(450, 300);
setResizable(true);
setLocationRelativeTo(null);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public void initOpenGL() {
// XXX: Client class should control when OpenGL gets initialized.
// Client may need to setup things OpenGL needs (e.g. read shader
// source) before it can get initialized to avoid crashes.
canvas = new GLCanvas();
canvas.addGLEventListener(this);
getContentPane().add(canvas);
}
}