Seeking Help with OpenGL Depth Buffer Issue
Posted by
xghost on
Sep 27, 2014; 7:55am
URL: https://forum.jogamp.org/Seeking-Help-with-OpenGL-Depth-Buffer-Issue-tp4033219.html
Hello,
I'm currently having a problem where I seem to be doing something
incorrectly, but I've not been able to identify the cause. (It might
be something silly I've overlooked...) Basically, I'm drawing
several objects onto a scene, but the objects show up in the order
in which they're rendered (i.e. last one to render shows in front).
See picture below for example. I'm using the latest release of JOGL
(Sept. 2014), too.
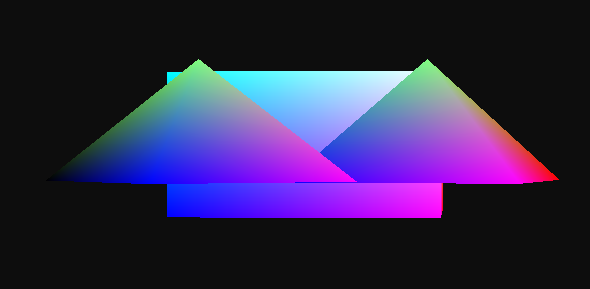
On the code side, I have depth testing enabled and I'm clearing it
before drawing the frame. Some of the code is below:
@Override
public void init(GLAutoDrawable auto) {
auto.setGL(new TraceGL4(new
DebugGL4(auto.getGL().getGL4()), System.out));
GL4 gl = (GL4) auto.getGL();
gl.glEnable(GL4.GL_CULL_FACE);
gl.glFrontFace(GL4.GL_CW);
gl.glEnable(GL4.GL_DEPTH_TEST);
gl.glDepthFunc(GL4.GL_LEQUAL);
// NOTE: Using GL_EQUAL above still
draws frame contents,
// but using GL_LESS shows no objects; it's as if all
pixels
// have the same depth value and so they always
get written
// keeping/removing these 3 makes no difference
gl.glDepthRange(0.0f, 1.0f);
gl.glClearDepthf(1.0f);
gl.glDepthMask(true);
createShaderProgram(gl);
gl.glGenVertexArrays(vao.length, vao, 0);
gl.glBindVertexArray(vao[0]);
modelMatrixLoc =
gl.glGetUniformLocation(program.program(), "model_matrix");
viewMatrixLoc =
gl.glGetUniformLocation(program.program(), "view_matrix");
projMatrixLoc =
gl.glGetUniformLocation(program.program(), "proj_matrix");
timer.scheduleAtFixedRate(new AnimationTask(), 0,
ANIMATION_DELAY);
}
The DebugGL4 trace shows that no failures or error reports. One
difference between my code and other code samples is that, since I'm
rendering different objects, I have different VBOs. The code to
render a single frame follows:
@Override
public void display(GLAutoDrawable auto) {
GL4 gl = (GL4) auto.getGL();
gl.glClearBufferfv(GL4.GL_COLOR, 0, clearColor);
gl.glClearBufferfv(GL4.GL_DEPTH, 0, clearDepth);
sun.rotate(0.5f, UP_AXIS);
double dt = System.currentTimeMillis() / 950.0;
double dx = Math.sin(2.1f * dt) * 0.5f;
// double dy = Math.cos(1.7f * dt) * 0.5f;
double dz = Math.sin(1.3f * dt) * Math.cos(1.5f * dt) *
0.5f;
planet1.getTranslation().setToIdentity();
planet2.getTranslation().setToIdentity();
planet1.translate( 0.25, 0.1, -0.5);
planet2.translate(-0.25, 0.1, -0.5);
planet1.rotate( 2.0, UP_AXIS);
planet2.rotate(-4.0, UP_AXIS);
gl.glUseProgram(program.program());
Matrix3D viewMatrix =
MathUtils.createLookAtMatrix(camera.getLocation(),
new Point3D(camera.getViewDirection()),
UP_AXIS);
gl.glUniformMatrix4fv(viewMatrixLoc, 1, false,
viewMatrix.getFloatValues(), 0);
gl.glUniformMatrix4fv(projMatrixLoc, 1, false,
projMatrix.getFloatValues(), 0);
render(auto, sun);
render(auto, planet1);
render(auto, planet2);
}
private void render(GLAutoDrawable auto, final Shape3D obj)
{
GL4 gl = (GL4) auto.getGL();
gl.glUniformMatrix4fv(modelMatrixLoc, 1, false,
obj.getTransform().getFloatValues(), 0);
obj.draw(auto);
}
This is what the drawing method for the Shape3D objects looks as
follows:
private void init(GL4
gl) {
// @formatter:off
final float[] vertices = new float[] {
// x, y, z vertex data here (the W component
defaults to 1 in the vertex shader)
};
// TODO: Create index buffer
// @formatter:on
vertexCount = vertices.length / 3;
gl.glGenBuffers(vbo.length, vbo, 0);
gl.glBindBuffer(GL4.GL_ARRAY_BUFFER, vbo[0]);
FloatBuffer buffer = FloatBuffer.wrap(vertices);
gl.glBufferData(GL4.GL_ARRAY_BUFFER, buffer.limit() * 3,
buffer, GL4.GL_STATIC_DRAW);
initialized = true;
}
@Override
public void draw(GLAutoDrawable auto) {
GL4 gl = (GL4) auto.getGL();
if (!initialized)
init(gl);
gl.glBindBuffer(GL4.GL_ARRAY_BUFFER, vbo[0]);
gl.glVertexAttribPointer(0, 3, GL4.GL_FLOAT, false, 0,
0);
gl.glEnableVertexAttribArray(0);
gl.glDrawArrays(GL4.GL_TRIANGLES, 0, vertexCount);
}
The objects are at known positions:
- Cube is at origin (0, 0, 0)
- L-Pyramid: (-0.25, 0.1, -0.5)
- R-Pyramid: (0.25, 0.1, -0.5)
They should both be getting covered by the cube, but that's clearly
not happening. I'm still not sure what I may be doing wrong and,
hopefully, someone can help me out with a fresh set of eyes. I can
share more code if necessary, but these seemed to be the more
relevant parts. Printing the capability data, I get:
GLCapabilities caps = new
GLCapabilities(GLProfile.getDefault(GLProfile.getDefaultDevice()));
System.out.println("Caps: " + caps.toString());
--- output ---
Caps: GLCaps[rgba 8/8/8/0, opaque, accum-rgba 0/0/0/0, dp/st/ms
16/0/0, dbl, mono , hw, GLProfile[GL4bc/GL4bc.hw], on-scr[.]]
The video card is nvidia gtx 770i on driver 331.38 on Kubuntu 14.04
AMD64.
Thanks for the help in advance.