Hello. I'm struggling with my textures and I would really appreciate some help.
I have a piece of code which renders a texture from a jpg file. The code gives different result for Linux and Mac and I can't understand if I am the cause for the problem (probably yes). On both machines I'm using Java 7 and the latest jogl libraries (since December 2013).
The code is giving me the results that I want on the Linux Machine. On the Mac, however, if the animator is running the image shows for a second and then it shrinks to an invisible size. If I don't start the animator the image stays but is smaller that the square that I'm trying to map it onto. I am inerting screenshots of what the results look like.
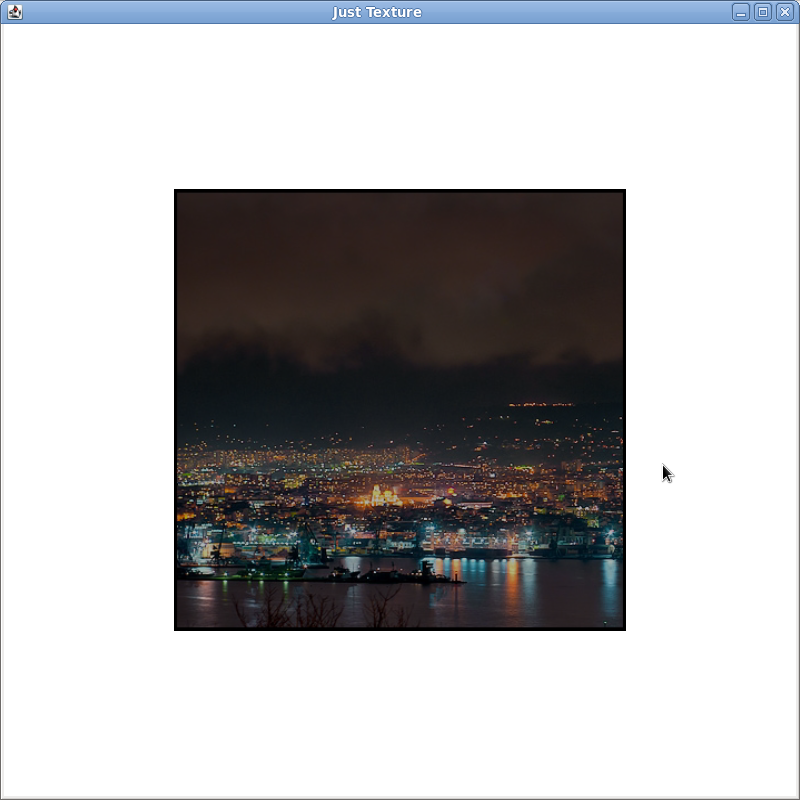
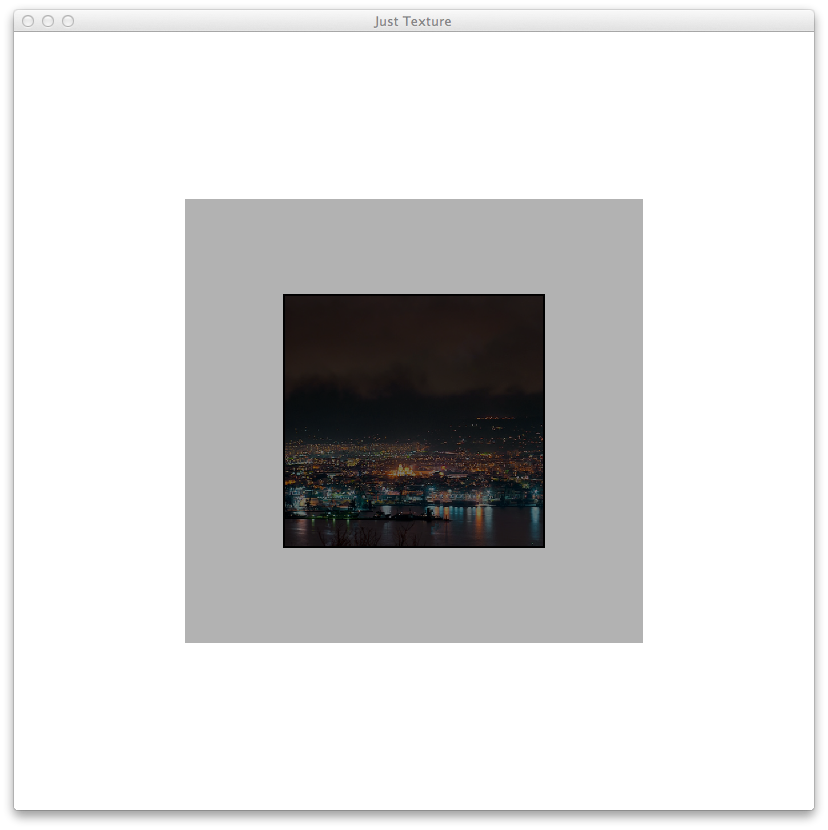
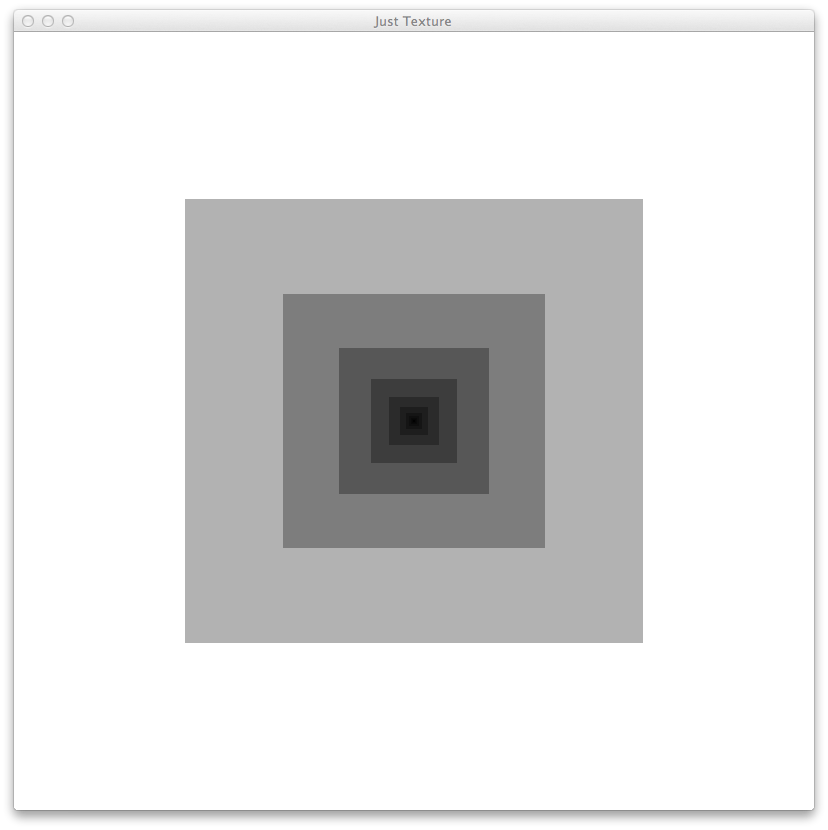
I am also attaching my code (and the 2 shaders). I think it's simple enough. The java class is
justTexture.java, the vertex shader
colorTexture.vert and the fragment shader
colorTexture.frag.
I suspect that maybe I'm doing something wrong when I activate and bind the texture or when I send the sampler2D location to the shader.
This is where I initializa the texture
texture = TextureIO.newTexture(new File("Varna.jpg"), false);
byte[] buf = new byte[texture.getWidth()*texture.getHeight()*4];
ByteBuffer buffer = ByteBuffer.wrap(buf);
gl.glGenTextures(1, textureId, 0);
gl.glActiveTexture(GL3.GL_TEXTURE0);
gl.glBindTexture(GL3.GL_TEXTURE_2D, textureId[0]);
gl.glTexImage2D(GL3.GL_TEXTURE_2D, 0, GL3.GL_RGBA, texture.getWidth(), texture.getHeight(), 0, GL3.GL_RGBA, GL3.GL_UNSIGNED_BYTE, buffer);
texture.setTexParameteri(gl, GL3.GL_TEXTURE_MIN_FILTER, GL3.GL_LINEAR);
texture.setTexParameteri(gl, GL3.GL_TEXTURE_MAG_FILTER, GL3.GL_LINEAR);
then I create a location for the sampler
iSampler = gl.glGetUniformLocation(iTexProgram, "Tex1");
and the code below goes in the display function
gl.glUseProgram(iTexProgram);
gl.glUniformMatrix4fv(iTexMVP, 1, false, texMVP, 0);
gl.glUniform1i(iSampler, 0);
gl.glBindVertexArray(iVao[0]);
gl.glDrawArrays(GL3.GL_TRIANGLES, 0, 6);
gl.glBindVertexArray(0);
Thank you in advance for your help. I really appreciate it.